Key Takeaways:
- Effective management of if statements is essential for clean and maintainable Python code.
- Expertise in ending if statements in Python can significantly improve coding skills for developers of all levels.
- Techniques for ending if statements include using else clauses, elif clauses, break statements, return statements, and raise statements.
- The else clause executes when the condition in the if statement is false, providing an alternative block of code to execute.
- Elif clauses offer a cleaner alternative to nested if statements for handling multiple conditions.
- The break statement exits a loop when a condition is met, effectively ending the if statement.
- The return statement exits a function and can be used within an if statement to conditionally exit a function.
- The raise statement raises exceptions to handle specific errors or exceptional cases, effectively halting the program execution.
- Understanding the basics of conditional logic in Python is crucial for effectively utilizing if statements in code.
Different scenarios may call for different techniques to conclude if statements, depending on the specific requirements of the program.
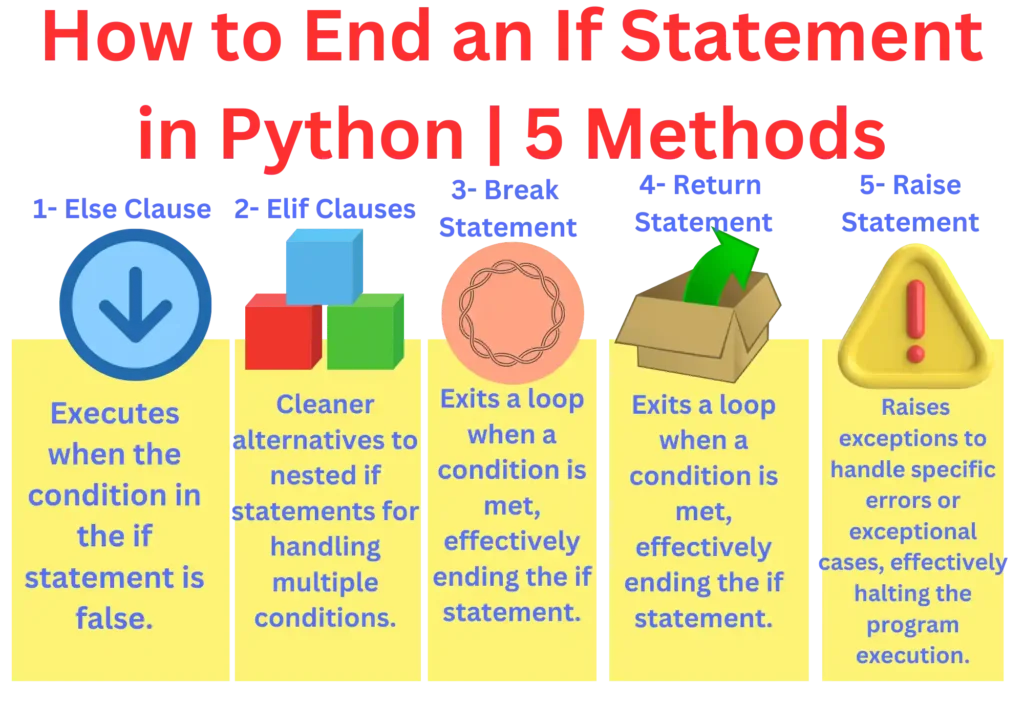
In Python programming, the effective management of if statements is crucial for writing clean and maintainable code. These conditional constructs allow you to control the flow of execution based on specified conditions.
Whether you are a starting or an experienced developer expertise in how to end an if statement in Python can significantly enhance your coding skills.
In this comprehensive guide we will discuss detailed different techniques how to end an if statement in Python:
- Using the else clause: The else block is executed when the condition in the if statement evaluates to false.
- Employing elif clauses: These are used for scenarios involving multiple conditions providing cleaner alternatives to nested if statements.
- Break statement: Used within loops it exits the loop when a condition is met effectively ending the if statement.
- Return statement: Ends the execution of a function and returns a value if specified effectively exiting the if statement as well.
- Raise statement: Raises an exception when a condition is met which can be used to exit the if statement and handle exceptional cases.
Table of Contents
ToggleUnderstanding the Basics
Picture your code as a series of instructions with if statements acting as checkpoints along the way. Python evaluates whether certain conditions hold true and executes the corresponding block of code within the if statement. If the condition is false and an else block is present Python executes the instructions within the else block. For scenarios involving multiple conditions you can employ elif clauses as cleaner alternatives to nested if statements.
Various Methods to Conclude an If Statement in Python
Basic Flow and else Clause
The easiest way to terminate an if statement is either allow the code run naturally after the if block or use else clause to handle the false condition.
For example:
age = 20
if age >= 18:
print("You can watch the movie or drama")
else:
print("Sorry you are too young for this type of movie or drama")
Using elif Clauses
elif clauses provide a straightforward way to check multiple conditions one after the other following the initial if statement.
For example:
age = 20
if age > 60:
print("You qualify for the senior discounts")
elif age >= 18:
print("You are an the adult now")
else:
print("You are still a the teenager")
Python evaluates each condition step by step and prints the appropriate message based on the given age.
Using the break Statement
Though primarily used with loops, the break statement can also exit an if statement early particularly within loops.
For instance:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
if num == 3:
print("Number 3 found!")
break
When Python comes across the number 3 it prints “Number 3 found!” and stops the loop immediately.
Using the return Statement
The return statement exits a function and can optionally return a value. It can be used within an if statement to exit a function conditionally.
For example:
def is_even(num):
if num % 2 == 0:
return True
return False
If the number is even the function returns Truly instantly; otherwise it moves to the next return statement.
Using the raise Statement
The raise statement raises exceptions in Python. It can be employed within an if statement to handle specific errors or exceptional cases.
For example:
x = -1
if x < 0:
raise ValueError("Number must be the positive +ve")
If x is negative Python raises a ValueError exception and halts the program execution.
Understanding How to End an If Statement in Python is crucial for writing concise and effective code. By mastering these methods, you can confidently handle conditional logic ensuring smooth execution of your programs in various scenarios. Whether you are new to the programming or an experienced coder these techniques enable you to utilize Python’s conditional constructs efficiently enhancing your coding skills as you progress.
Recommneded Article: