You can learn how to make a basic Python Convert Feet to Meters Code in this guide. For learners, teachers, and anyone who wants to carry out this common conversion quickly, this is a useful ability. The approach is as follows:
- Start from scratch: Assuming you have never programmed before, we will begin with the fundamentals (basic).
- Step-by-Step: We’re going to divide the process into practical, simple and easy steps.
- Python Code Snippet: By the time (timestamp) you have completed this article, you will have a useful Python code snippet that you are able to share to others.
Table of Contents
ToggleBuilding the Python Code Step by Step
Step 1: Understanding the Conversion
The first thing to know in our coding journey is the secret conversion code:
- 1 foot equals 0.3048 meters. (1Foot= 0.3048)
- Think of it like a special decoder ring that helps us translate between different units.
Step 2: Creating the Conversion Function
The next step is to unleash our imagination and create the “feet_to_meters” Python function. Our superhero will be this function, taking care of all the tedious job for us.
- It takes a measurement in feet.
- Magically transforms it into meters.
No need to think about the specifics of the conversion process; just picture it as a magical wand that you can wave to make it happen.
def feet_to_meters(feet):
# 1 foot is approximately 0.3048 meters
meters = feet * 0.3048
return meters
Step 3: Getting Your Measurement
Suppose that you are in a school or some other situation where you need to convert measurements. You will begin by requesting the length in feet. For this section, we’ll use Python’s input function:
feet_str = input(“Please enter the length in feet: “)
Step 4: Handling User Input
Errors occur, especially when you’re rushing. For that, our Python code is ready! It can manage two different situations:
- If you enter a valid number, the conversion will start.
- It will carefully inform you of the error if you mistakenly type something other than a number (such as characters,symbols, words, elements).
The code to implement this is as follows:
try:
feet = float(feet_str)
# Check if the input is non-negative
if feet >= 0:
# Time to convert! We’ll call our conversion function.
meters = feet_to_meters(feet)
# And now, we’ll display the result for you.
print(f”{feet} feet is roughly {meters:.2f} meters”)
else:
print(“Please enter a non-negative number of feet.”)
except ValueError:
print(“Oops, that doesn’t look like a valid number. Please try again.”)
Step 5: Completing the Task
Copy and paste the entire code below and run it in your Python environment to use this useful converting tool in daily life. It’s going to work as if by magic!
Here is Free Online Compiler: Click Here to run Your Code
def feet_to_meters(feet):
# 1 foot is approximately 0.3048 meters
meters = feet * 0.3048
return meters
feet_str = input(“Please enter the length in feet: “)
try:
feet = float(feet_str)
if feet >= 0:
meters = feet_to_meters(feet)
print(f”{feet} feet is roughly {meters:.2f} meters”)
else:
print(“Please enter a non-negative number of feet.”)
except ValueError:
print(“Oops, that doesn’t look like a valid number. Please try again.”)
How to Use the Code
- Copy the code above.
- Open your Python environment (IDLE, Jupyter Notebook, or any Python interpreter).
- Paste the code into the Python environment.
- Run the code.
- Enter the length in feet when prompted.
- The code will calculate and display the equivalent length in meters.
Output Screen Shot
Suppose you want to convert 5 feet into meters. Enter “5” when prompted, and the code will display the result as follows:
Enter the length in feet: 5
5.0 feet is equal to 1.52 meters
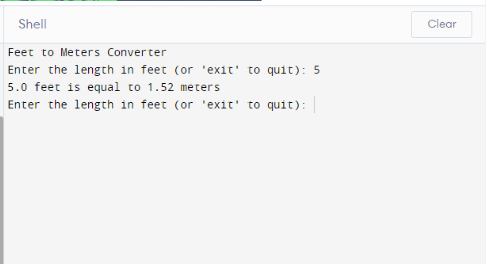
Programmiz Python Online Compiler: Click Here To Run Your Python Code
Conclusion
The guide tutorial post article offers a step-by-step tutorial for writing Python code to convert feet to meters. It divides the procedure into the following 5 steps:
- Starting from scratch with basic programming concepts.
- Taking a step-by-step approach to simplify the conversion.
- Creating a Python code snippet for easy sharing.
- Understanding the conversion factor (1 foot = 0.3048 meters).
- Developing a Python function for the conversion and handling user input.
The piece of writing article post ends by showing how to use the code while highlighting its usefulness and simplicity or clarity. To easily convert from feet to meters, users can simply copy, paste, and run the provided code in a Python environment. It is a useful tool for everyday conversions because sample usage is also shown.
People Also Read and Search:
FAQs:
What is the code to convert feet to meters?
The Python code to convert feet to meters is as follows:
def feet_to_meters(feet):
# 1 foot is approximately 0.3048 meters
meters = feet * 0.3048
return meters
How do you convert feet to centimeters in Python?
To convert feet to centimeters in Python, you can use the formula: 1 foot equals 30.48 centimeters. You can create a Python function similar to the one used for meters, replacing the conversion factor with 30.48.
How do you convert meters to inches in Python?
To convert meters to inches in Python, you can use the formula: 1 meter equals 39.37 inches. Create a Python function that takes a measurement in meters and multiplies it by 39.37 to get the equivalent length in inches.
What is the formula of M to feet?
The formula to convert meters (M) to feet (ft) is: 1 meter (M) equals approximately 3.28084 feet (ft).
What is 2 feet in meters?
2 feet is equal to approximately 0.6096 meters. You can use the Python code snippet provided earlier to perform this conversion.
How do you write 4 feet in cm?
To convert 4 feet to centimeters (cm), you can use the formula: 1 foot equals 30.48 centimeters. So, 4 feet is equal to 4 x 30.48 = 121.92 centimeters.
How do you convert feet to meters manually?
Manually converting feet to meters involves multiplying the length in feet by the conversion factor, which is approximately 0.3048. For example, to convert 5 feet to meters manually, you would calculate: 5 feet x 0.3048 ≈ 1.524 meters.